How to Center an Image with CSS
Introduction
Some design layouts demand images to be perfectly aligned, either horizontally, vertically, or a mix of both. This guide offers a comprehensive walk-through with examples covering a range of such scenarios.
Dealing with images can often be a challenging task. To gain a better understanding of their behaviour, here are some key considerations.
Images are Inline by Default
Images serve as content, similar to text, and are treated as such by default.
This is why the display: inline
rule applies.
Intrinsic Dimensions of an Image
Consider an image with the dimensions 150px
x 150px
.
Unless specific CSS is applied to alter these dimensions, the image will retain its original size upon embedding.
Text-align
If the parent element of an image is a block-level element, you can align the image horizontally by just applying text-align: center
on the parent.
In the example below the body element is the parent of the image.
Browsers apply display: block
to it by default, you can confirm this by inspecting the code with developer tools.
See what happens when you remove the comment from the display: inline
rule, you'll notice that the image ceases to be center-aligned.
This will work horizontally, but what if you want to place an image both vertically and horizontally?
Absolute positioning
For me this method is a thing of the past, but you never know there may be the odd occasion where you are working with some constraints and this is the only way to go about doing the job, so for completeness here it is:
This involves applying position: relative
to the parent, which in this case is a <div>
element, then you can use absolute positioning on the image.
To explain a bit more about why different positioning is required on a parent, check out the Mozilla docs:
The element is removed from the normal document flow, and no space is created for the element in the page layout. It is positioned relative to its closest positioned ancestor, if any; otherwise, it is placed relative to the initial containing block. Its final position is determined by the values of top, right, bottom, and left.
This technique won't work without also applying the transform property. The combination of top: 50%
, right: 50%
, bottom: 50%
, left: 50%
and transform: translate(-50%,-50%)
allows the intrinsic size of the image to be accounted for, if you go ahead and change th size of the image you'll see it's still in the center.
Margin auto
Similarly to text-align, this is another case where you can align an image horizontally, but with a caveat:
At the start of the guide I mention:
Images Are Inline by Default
margin: auto
won't work on inline elements, therefore the image requires its display property to be amended.
Flexbox
If you read the centering content section in my CSS Grid VS Flexbox guide, you'll already be familiar with the following approach.
By wrapping the image with an element which has display: flex
applied to it changes the behaviour of its children (flex-items), allowing you to align content both horizontally and vertically.
Combining justify-content
and align-items
retains the intrinsic dimensions of the image.
What happens to the image if you remove align-items
in the code playground?
The image fills the available space.
Ultimately this is down to flexbox being one dimensional, and without getting into the internal mechanisms of how flexbox works, it feels unintuitive when compared to the next example.
CSS Grid
The centering content section also covers CSS Grid, go check it out if you haven't already.
CSS Grid is two dimensional, so you can apply the one-liner place-content: center
.
Again, similarly to Flexbox, if you apply display: grid
to the parent element, the image becomes a grid-item and you can then control it either by applying rules to the parent, or to the image itself.
And 💥 there you have it, it's that simple, by the way place-content: center
is shorthand for:
body {
align-content: center;
justify-content: center;
}
Conclusion
From the default inline nature of images to the power of CSS Grid, there are numerous ways to achieve your design goals.
Remember, CSS is a vast ocean of possibilities, and centering an image is just one small wave. Whether you opt for the classic text-align technique or choose to harness the modern prowess of Flexbox and CSS Grid, the ultimate key lies in understanding the inherent behaviors and limitations of each method.
If you have any suggestions as to how the examples can be made clearer, easier to understand, or how I can add any missing use-cases you feel would help others, I'd love nothing more than to hear from you! Reach out to me on Twitter, or shoot me an email.
Written by Morgan Feeney
I’ve been designing and developing for almost 2 decades.
Read about me, and my work.
For juicy tips and exclusive content, subscribe to my FREE newsletter.
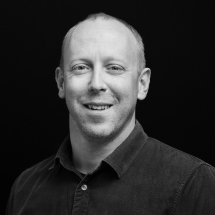